Template Strings
As of ES6, JS now has template strings as an alternative to the classic end quotes strings.
Ex: Normal string
var firstName = 'Jake';
var lastName = 'Rawr';
console.log('My name is ' + firstName + ' ' + lastName);
// My name is Jake Rawr
Template String
var firstName = 'Jake';
var lastName = 'Rawr';
console.log(`My name is ${firstName} ${lastName}`);
// My name is Jake Rawr
You can do multi-line strings without \n
, perform simple logic (ie 2+3) or even use the ternary operator inside ${}
in template strings.
var val1 = 1, val2 = 2;
console.log(`${val1} is ${val1 < val2 ? 'less than': 'greater than'} ${val2}`)
// 1 is less than 2
You are also able to modify the output of template strings using a function; they are called tagged template strings for example usages of tagged template strings.
You may also want to read to understand template strings more.
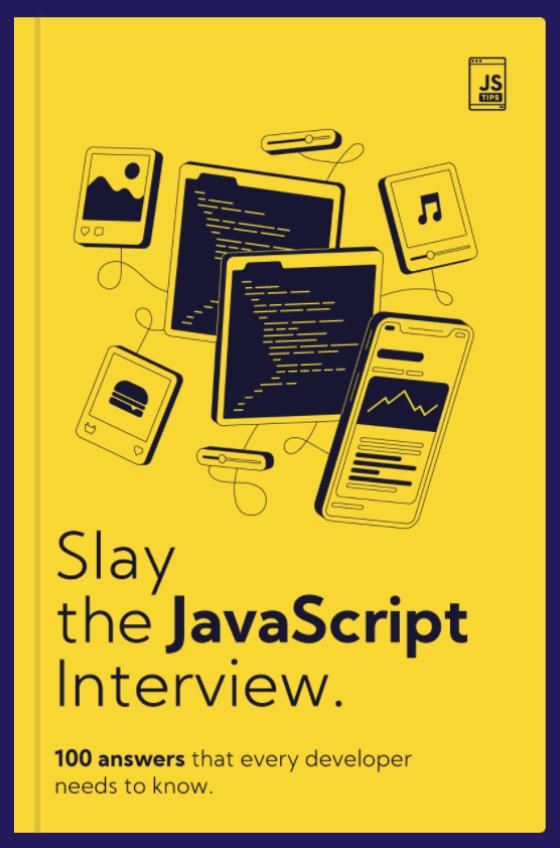
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
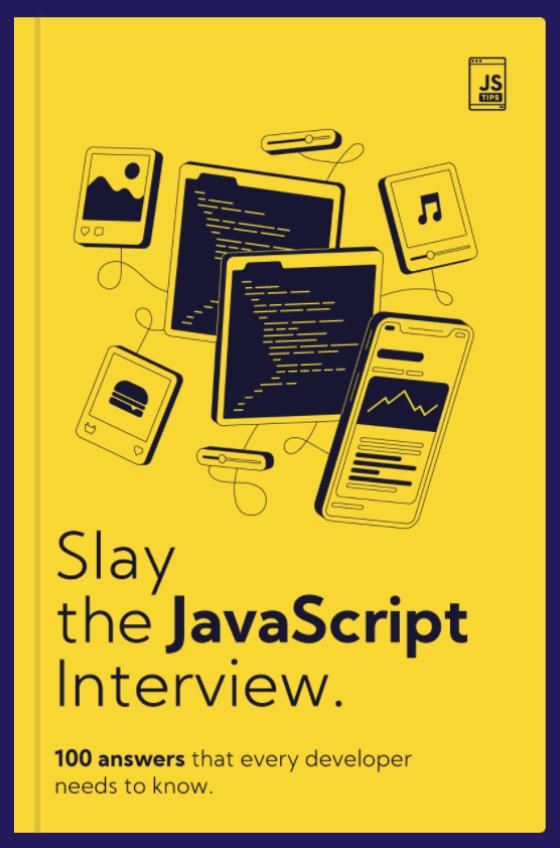
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW