Basics declarations
Below, different ways to declare variables in JavaScript. Comments and console.log should be enough to explain what’s happening here:
var y, x = y = 1 //== var x; var y; x = y = 1
console.log('--> 1:', `x = ${x}, y = ${y}`)
// Will print
//--> 1: x = 1, y = 1
First, we just set two variables. Nothing much here.
;(() => {
var x = y = 2 // == var x; x = y = 2;
console.log('2.0:', `x = ${x}, y = ${y}`)
})()
console.log('--> 2.1:', `x = ${x}, y = ${y}`)
// Will print
//2.0: x = 2, y = 2
//--> 2.1: x = 1, y = 2
As you can see, the code has only changed the global y, as we haven’t declared the variable in the closure.
;(() => {
var x, y = 3 // == var x; var y = 3;
console.log('3.0:', `x = ${x}, y = ${y}`)
})()
console.log('--> 3.1:', `x = ${x}, y = ${y}`)
// Will print
//3.0: x = undefined, y = 3
//--> 3.1: x = 1, y = 2
Now we declare both variables through var. Meaning they only live in the context of the closure.
;(() => {
var y, x = y = 4 // == var x; var y; x = y = 4
console.log('4.0:', `x = ${x}, y = ${y}`)
})()
console.log('--> 4.1:', `x = ${x}, y = ${y}`)
// Will print
//4.0: x = 4, y = 4
//--> 4.1: x = 1, y = 2
Both variables have been declared using var and only after that we’ve set their values. As local > global, x and y are local in the closure, meaning the global x and y are untouched.
x = 5 // == x = 5
console.log('--> 5:', `x = ${x}, y = ${y}`)
// Will print
//--> 5: x = 5, y = 2
This last line is explicit by itself.
You can test this and see the result thanks to babel.
More informations available on the MDN.
Special thanks to @kurtextrem for his collaboration :)!
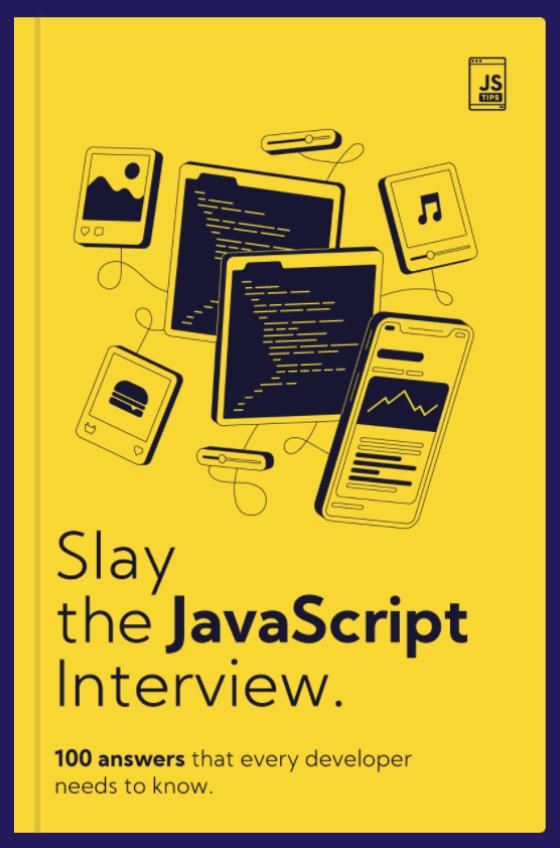
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
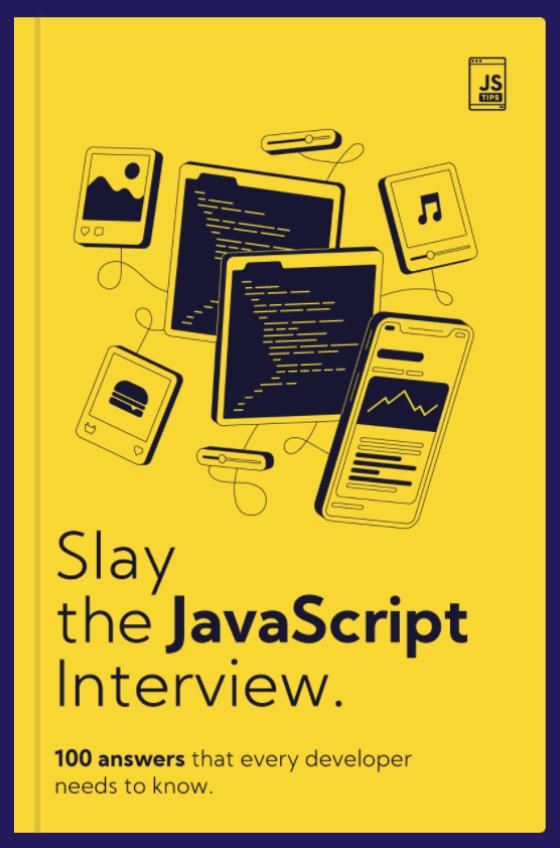
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW