Binding objects to functions
More than often, we need to bind an object to a function’s this object. JS uses the bind method when this is specified explicitly and we need to invoke desired method.
Bind syntax
fun.bind(thisArg[, arg1[, arg2[, ...]]])
Parameters
thisArg
this
parameter value to be passed to target function while calling the bound
function.
arg1, arg2, …
Prepended arguments to be passed to the bound
function while invoking the target function.
Return value
A copy of the given function along with the specified this
value and initial arguments.
Bind method in action in JS
const myCar = {
brand: 'Ford',
type: 'Sedan',
color: 'Red'
};
const getBrand = function () {
console.log(this.brand);
};
const getType = function () {
console.log(this.type);
};
const getColor = function () {
console.log(this.color);
};
getBrand(); // object not bind,undefined
getBrand(myCar); // object not bind,undefined
getType.bind(myCar)(); // Sedan
let boundGetColor = getColor.bind(myCar);
boundGetColor(); // Red
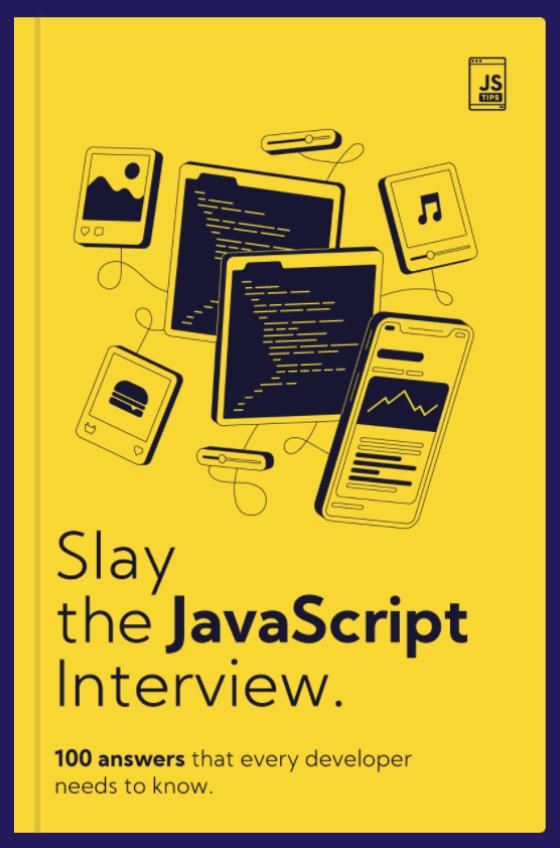
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
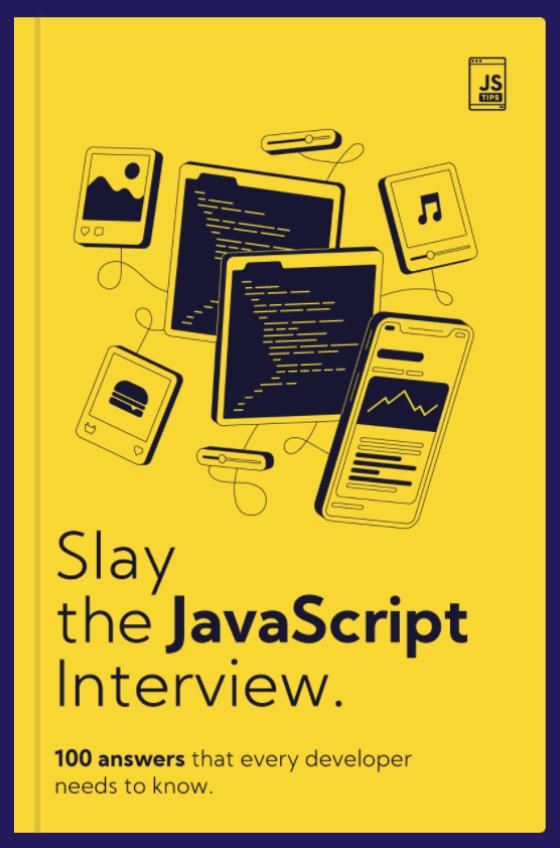
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW