Calculate the Max/Min value from an array
The built-in functions Math.max() and Math.min() find the maximum and minimum value of the arguments, respectively.
Math.max(1, 2, 3, 4); // 4
Math.min(1, 2, 3, 4); // 1
These functions will not work as-is with arrays of numbers. However, there are some ways around this.
Function.prototype.apply()
allows you to call a function with a given this
value and an array of arguments.
var numbers = [1, 2, 3, 4];
Math.max.apply(null, numbers) // 4
Math.min.apply(null, numbers) // 1
Passing the numbers
array as the second argument of apply()
results in the function being called with all values in the array as parameters.
A simpler, ES2015 way of accomplishing this is with the new spread operator.
var numbers = [1, 2, 3, 4];
Math.max(...numbers) // 4
Math.min(...numbers) // 1
This operator causes the values in the array to be expanded, or “spread”, into the function’s arguments.
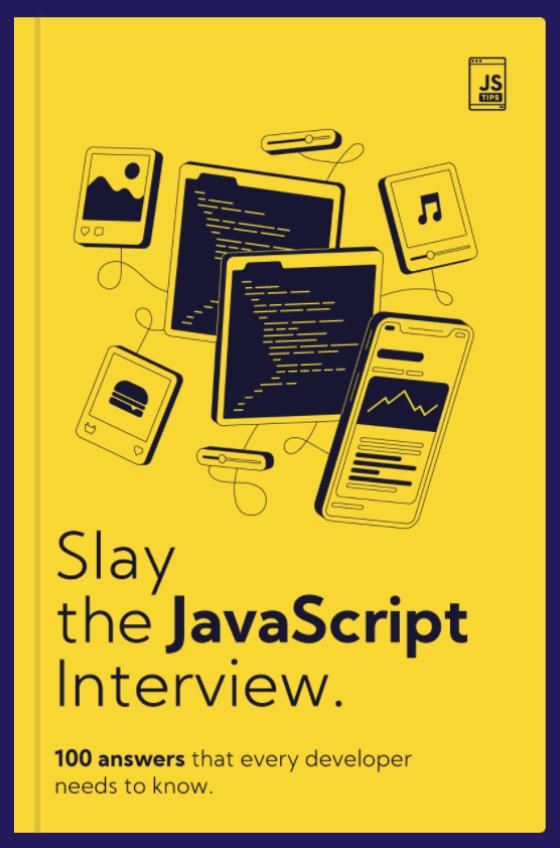
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
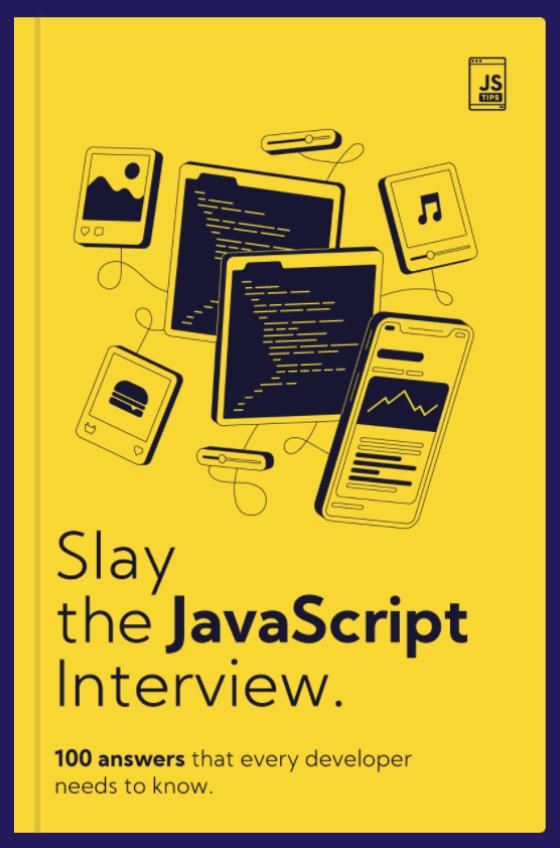
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW