Flattening multidimensional Arrays in JavaScript
These are the three known ways to merge multidimensional array into a single array.
Given this array:
var myArray = [[1, 2],[3, 4, 5], [6, 7, 8, 9]];
We wanna have this result:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Solution 1: Using concat()
and apply()
var myNewArray = [].concat.apply([], myArray);
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
Solution 2: Using reduce()
var myNewArray = myArray.reduce(function(prev, curr) {
return prev.concat(curr);
});
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
Solution 3:
var myNewArray3 = [];
for (var i = 0; i < myArray.length; ++i) {
for (var j = 0; j < myArray[i].length; ++j)
myNewArray3.push(myArray[i][j]);
}
console.log(myNewArray3);
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
Solution 4: Using spread operator in ES6
var myNewArray4 = [].concat(...myArray);
console.log(myNewArray4);
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
Solution 5: Using flat()
in ES10
var myNewArray5 = myArray.flat();
console.log(myNewArray5);
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
Take a look here these 4 algorithms in action.
For infinitely nested array try Lodash flattenDeep().
If you are curious about performance, here a test for check how it works.
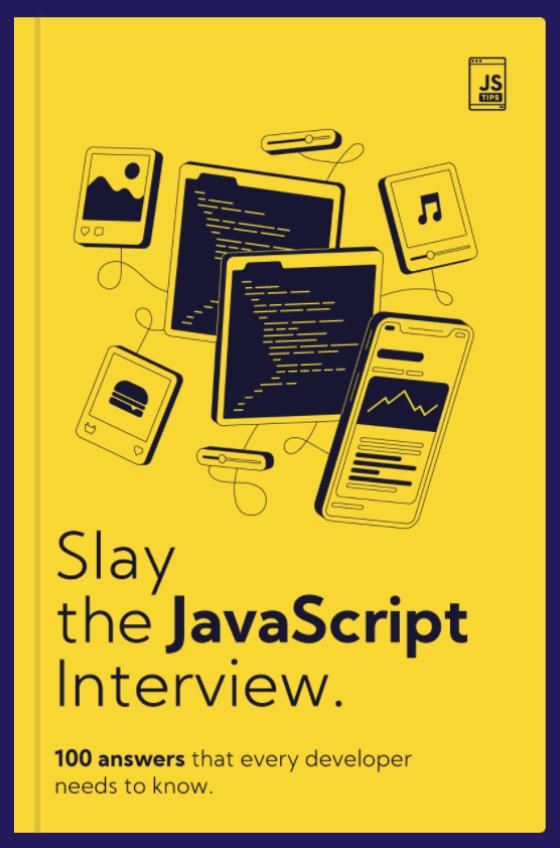
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
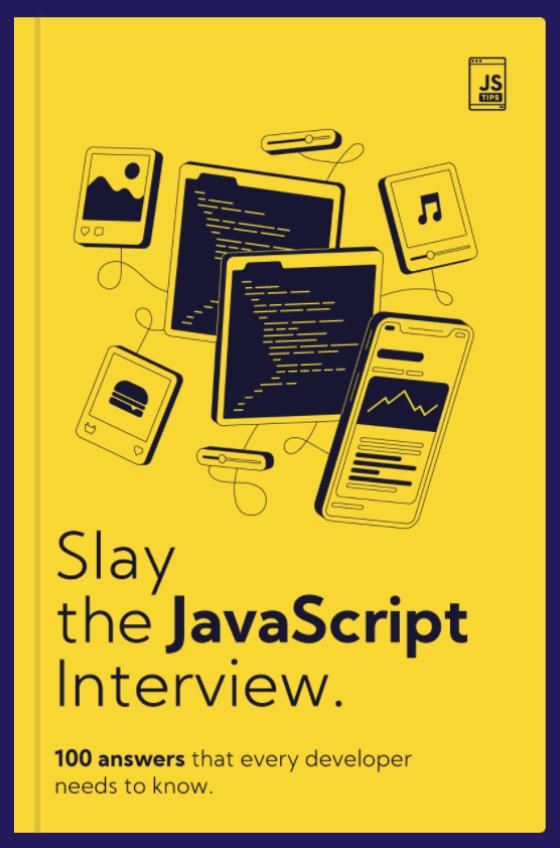
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW