Get File Extension
Question: How to get the file extension?
var file1 = "50.xsl";
var file2 = "30.doc";
getFileExtension(file1); //returs xsl
getFileExtension(file2); //returs doc
function getFileExtension(filename) {
/*TODO*/
}
Solution 1: Regular Expression
function getFileExtension1(filename) {
return (/[.]/.exec(filename)) ? /[^.]+$/.exec(filename)[0] : undefined;
}
Solution 2: String split
method
function getFileExtension2(filename) {
return filename.split('.').pop();
}
Those two solutions couldnot handle some edge cases, here is another more robust solution.
Solution3: String slice
, lastIndexOf
methods
function getFileExtension3(filename) {
return filename.slice((filename.lastIndexOf(".") - 1 >>> 0) + 2);
}
console.log(getFileExtension3('')); // ''
console.log(getFileExtension3('filename')); // ''
console.log(getFileExtension3('filename.txt')); // 'txt'
console.log(getFileExtension3('.hiddenfile')); // ''
console.log(getFileExtension3('filename.with.many.dots.ext')); // 'ext'
How does it works?
- String.lastIndexOf() method returns the last occurrence of the specified value (
'.'
in this case). Returns-1
if the value is not found. - The return values of
lastIndexOf
for parameter'filename'
and'.hiddenfile'
are-1
and0
respectively. Zero-fill right shift operator (»>) will transform-1
to4294967295
and-2
to4294967294
, here is one trick to insure the filename unchanged in those edge cases. - String.prototype.slice() extracts file extension from the index that was calculated above. If the index is more than the length of the filename, the result is
""
.
Comparison
Solution | Paramters | Results |
---|---|---|
Solution 1: Regular Expression | ’‘ ‘filename’ ‘filename.txt’ ‘.hiddenfile’ ‘filename.with.many.dots.ext’ |
undefined undefined ‘txt’ ‘hiddenfile’ ‘ext’ |
Solution 2: String split |
’‘ ‘filename’ ‘filename.txt’ ‘.hiddenfile’ ‘filename.with.many.dots.ext’ |
’’ ‘filename’ ‘txt’ ‘hiddenfile’ ‘ext’ |
Solution 3: String slice , lastIndexOf |
’‘ ‘filename’ ‘filename.txt’ ‘.hiddenfile’ ‘filename.with.many.dots.ext’ |
’’ ‘’ ‘txt’ ‘’ ‘ext’ |
Live Demo and Performance
Here is the live demo of the above codes.
Here is the performance test of those 3 solutions.
Source
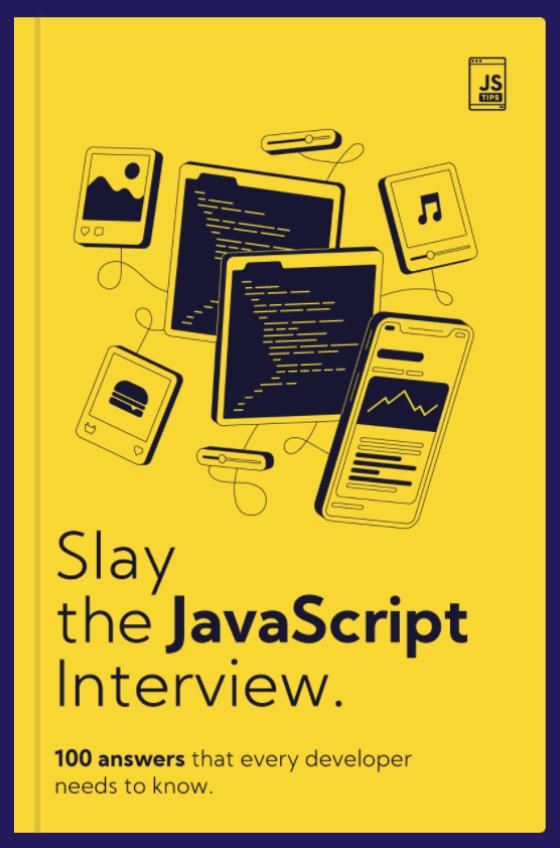
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
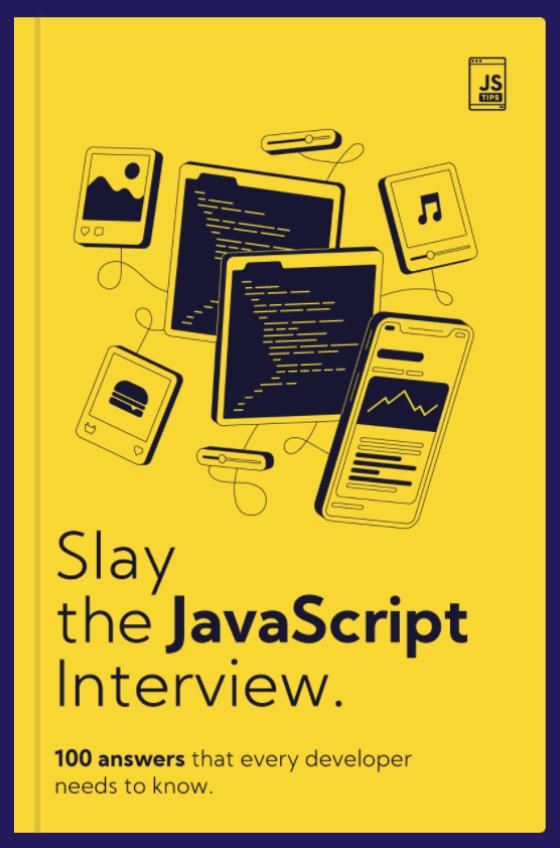
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW