Create an easy loop using an array
Sometimes, we need to loop endlessly over an array of items, like a carousel of images or an audio playlist. Here’s how to take an array and give it “looping powers”:
var aList = ['A','B','C','D','E'];
function make_looper( arr ){
arr.loop_idx = 0;
// return current item
arr.current = function(){
if( this.loop_idx < 0 ){// First verification
this.loop_idx = this.length - 1;// update loop_idx
}
if( this.loop_idx >= this.length ){// second verification
this.loop_idx = 0;// update loop_idx
}
return arr[ this.loop_idx ];//return item
};
// increment loop_idx AND return new current
arr.next = function(){
this.loop_idx++;
return this.current();
};
// decrement loop_idx AND return new current
arr.prev = function(){
this.loop_idx--;
return this.current();
};
}
make_looper( aList);
aList.current();// -> A
aList.next();// -> B
aList.next();// -> C
aList.next();// -> D
aList.next();// -> E
aList.next();// -> A
aList.pop() ;// -> E
aList.prev();// -> D
aList.prev();// -> C
aList.prev();// -> B
aList.prev();// -> A
aList.prev();// -> D
Using the %
( Modulus ) operator is prettier.The modulus return division’s rest ( 2 % 5 = 1
and 5 % 5 = 0
):
var aList = ['A','B','C','D','E'];
function make_looper( arr ){
arr.loop_idx = 0;
// return current item
arr.current = function(){
this.loop_idx = ( this.loop_idx ) % this.length;// no verification !!
return arr[ this.loop_idx ];
};
// increment loop_idx AND return new current
arr.next = function(){
this.loop_idx++;
return this.current();
};
// decrement loop_idx AND return new current
arr.prev = function(){
this.loop_idx += this.length - 1;
return this.current();
};
}
make_looper( aList);
aList.current();// -> A
aList.next();// -> B
aList.next();// -> C
aList.next();// -> D
aList.next();// -> E
aList.next();// -> A
aList.pop() ;// -> E
aList.prev();// -> D
aList.prev();// -> C
aList.prev();// -> B
aList.prev();// -> A
aList.prev();// -> D
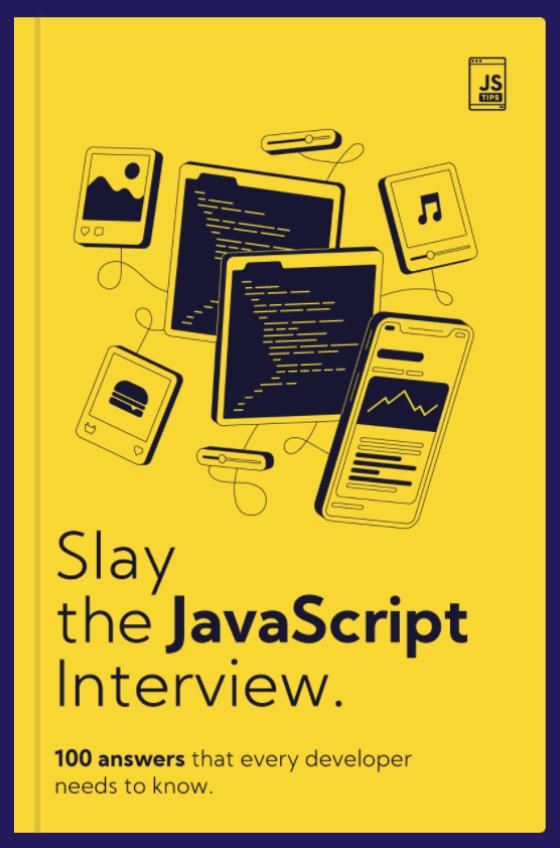
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
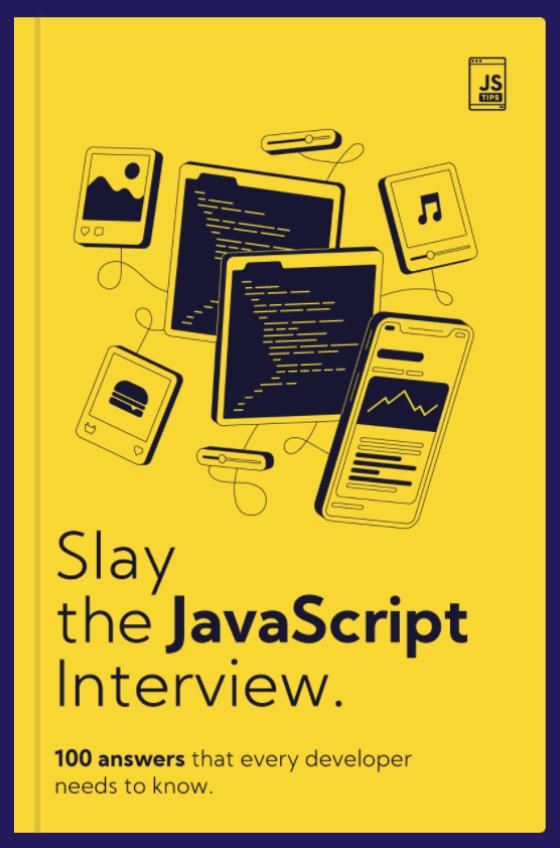
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW