What is a currying function?
A currying function is a function that takes multiple arguments and turns it into a sequence of functions having only one argument at a time.
In this way, an n-ary function becomes a unary function, and the last function returns the result of all the arguments together in a function.
// Normal definition
function multiply(a, b, c) {
return a * b * c;
};
console.log(multiply(1, 2, 3));
// Output: 6
// Simple curry function definition
function multiply(a) {
return (b) => {
return (c) => {
return a * b * c;
};
};
};
console.log(multiply(1)(2)(3));
// Output: 6
Further readings:
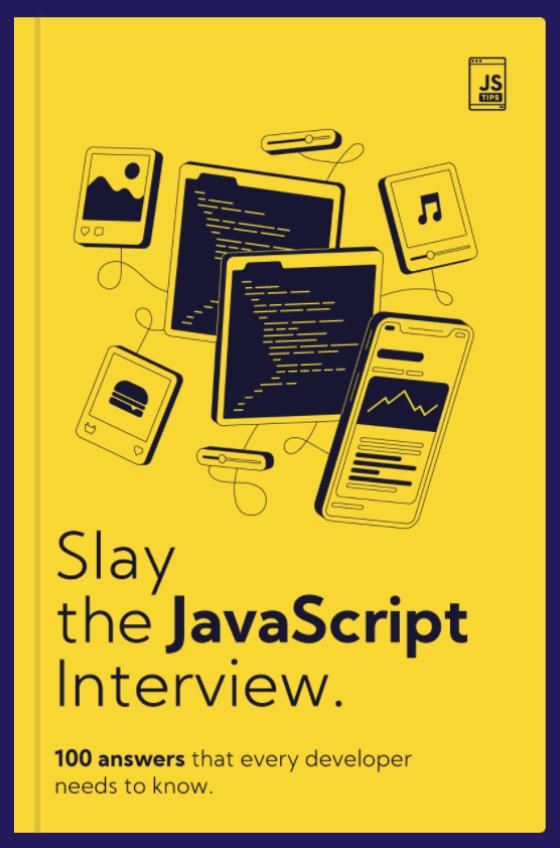
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
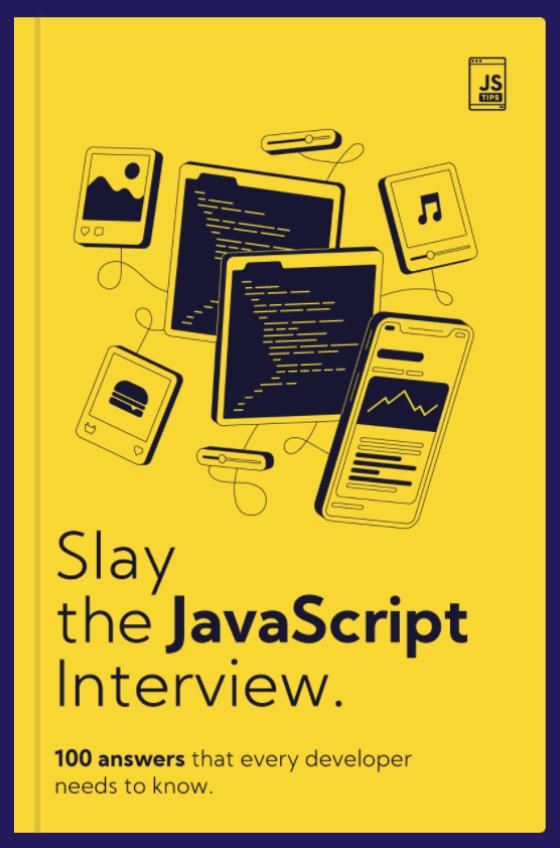
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW