What is Functional Inheritance?
Functional inheritance is the process of inheriting features by applying an augmenting function to an object instance. The function supplies a closure scope which you can use to keep some data private. The augmenting function uses dynamic object extension to extend the object instance with new properties and methods.
Functional mixins are composable factory functions that add properties and behaviors to objects like stations in an assembly line.
// Base object constructor function
function Animal(data) {
var that = {}; // Create an empty object
that.name = data.name; // Add it a "name" property
return that; // Return the object
};
// Create achild object, inheriting from the base Animal
function Cat(data) {
// Create the Animal object
var that = Animal(data);
// Extend base object
that.sayHello = function() {
return 'Hello, I\'m ' + that.name;
};
return that;
};
// Usage
var myCat = Cat({ name: 'Rufi' });
console.log(myCat.sayHello());
// Output: "Hello, I'm Rufi"
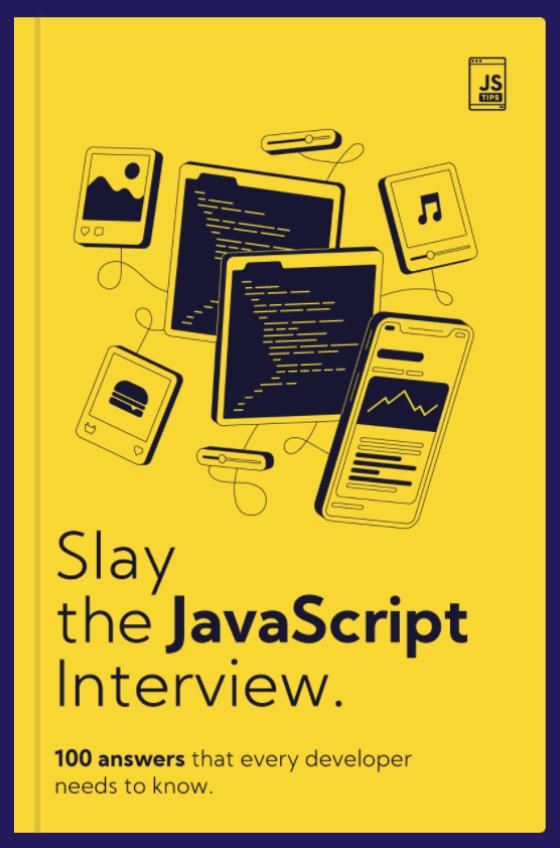
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
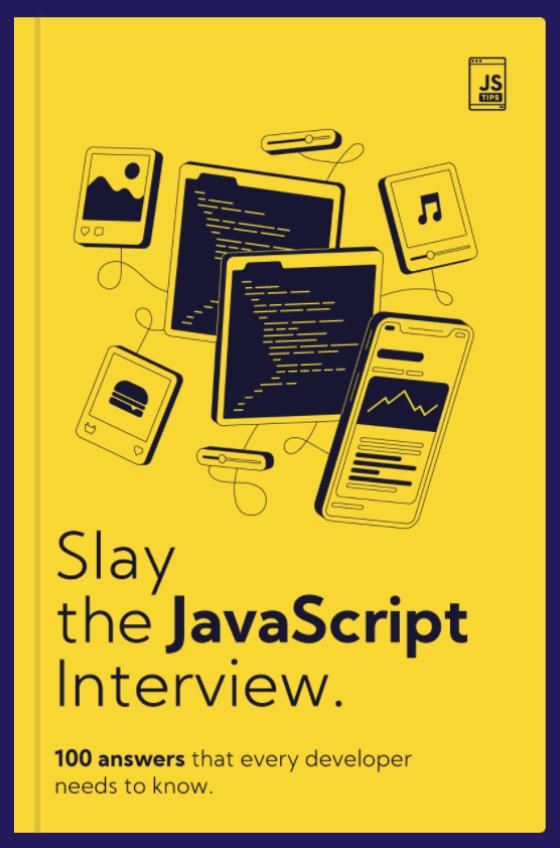
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW