用数组建立一个简单的循环
有时我们需要不停的循环数组的元素,就像一组旋转的图片,或者音乐的播放列表。这里告诉你如何使一个数组拥有循环的能力:
var aList = ['A','B','C','D','E'];
function make_looper( arr ){
arr.loop_idx = 0;
// 返回当前的元素
arr.current = function(){
if( this.loop_idx < 0 ){// 第一次检查
this.loop_idx = this.length - 1;// 更新 loop_idx
}
if( this.loop_idx >= this.length ){// 第二次检查
this.loop_idx = 0;// 更新 loop_idx
}
return arr[ this.loop_idx ];//返回元素
};
// 增加 loop_idx 然后返回新的当前元素
arr.next = function(){
this.loop_idx++;
return this.current();
};
// 减少 loop_idx 然后返回新的当前元素
arr.prev = function(){
this.loop_idx--;
return this.current();
};
}
make_looper( aList);
aList.current();// -> A
aList.next();// -> B
aList.next();// -> C
aList.next();// -> D
aList.next();// -> E
aList.next();// -> A
aList.pop() ;// -> E
aList.prev();// -> D
aList.prev();// -> C
aList.prev();// -> B
aList.prev();// -> A
aList.prev();// -> D
使用 %
( 取模 ) 操作符更优雅。取模返回除法的余数 ( 2 % 5 = 1
and 5 % 5 = 0
):
var aList = ['A','B','C','D','E'];
function make_looper( arr ){
arr.loop_idx = 0;
// return current item
arr.current = function(){
this.loop_idx = ( this.loop_idx ) % this.length;// 无需检查 !!
return arr[ this.loop_idx ];
};
// 增加 loop_idx 然后返回新的当前元素
arr.next = function(){
this.loop_idx++;
return this.current();
};
// 减少 loop_idx 然后返回新的当前元素
arr.prev = function(){
this.loop_idx += this.length - 1;
return this.current();
};
}
make_looper( aList);
aList.current();// -> A
aList.next();// -> B
aList.next();// -> C
aList.next();// -> D
aList.next();// -> E
aList.next();// -> A
aList.pop() ;// -> E
aList.prev();// -> D
aList.prev();// -> C
aList.prev();// -> B
aList.prev();// -> A
aList.prev();// -> D
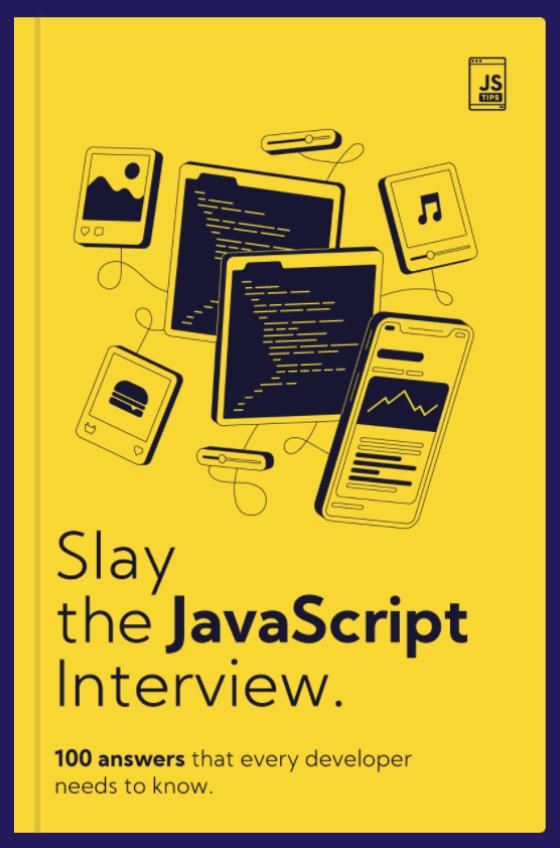
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
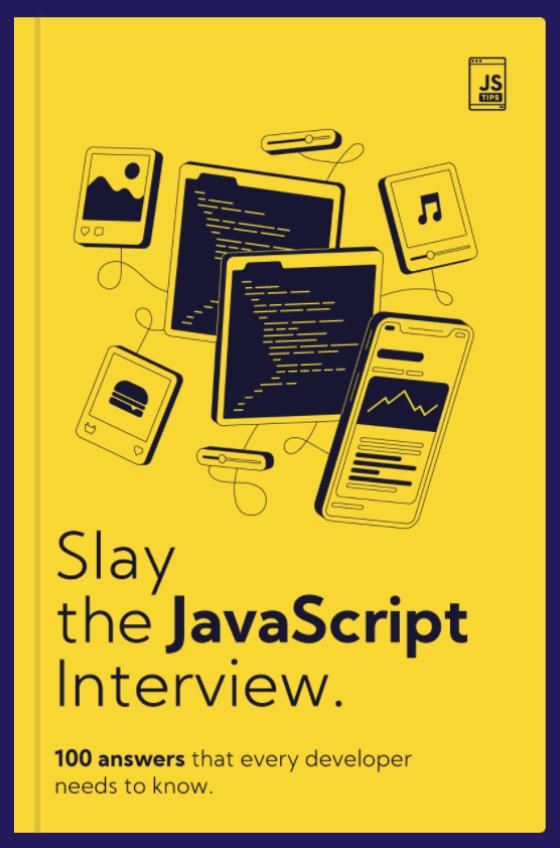
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW