宣告的基本知識
以下有不同的 JavaScript 變數宣告方式。
註解和 console.log
應該可以足夠解釋在這裡發生了什麼:
var y, x = y = 1 //== var x; var y; x = y = 1
console.log('--> 1:', `x = ${x}, y = ${y}`)
// 將會印出
//--> 1: x = 1, y = 1
首先,我們只是設定兩個變數,沒有其他的變數。
;(() => {
var x = y = 2 // == var x; x = y = 2;
console.log('2.0:', `x = ${x}, y = ${y}`)
})()
console.log('--> 2.1:', `x = ${x}, y = ${y}`)
// 將會印出
//2.0: x = 2, y = 2
//--> 2.1: x = 1, y = 2
你可以看到,程式碼只改變了全域的 y,因為我們還沒有宣告變數在 closure。
;(() => {
var x, y = 3 // == var x; var y = 3;
console.log('3.0:', `x = ${x}, y = ${y}`)
})()
console.log('--> 3.1:', `x = ${x}, y = ${y}`)
// 將會印出
//3.0: x = undefined, y = 3
//--> 3.1: x = 1, y = 2
現在我們透過 var 來宣告兩個變數。意思說它們只存在 closure 的 context。
;(() => {
var y, x = y = 4 // == var x; var y; x = y = 4
console.log('4.0:', `x = ${x}, y = ${y}`)
})()
console.log('--> 4.1:', `x = ${x}, y = ${y}`)
// 將會印出
//4.0: x = 4, y = 4
//--> 4.1: x = 1, y = 2
兩個變數已經使用 var 宣告,也設定了他們的值。既然 local > global
,x 和 y 在 closure 內,意思說全域的 x 和 y 是不會改變的。
x = 5 // == x = 5
console.log('--> 5:', `x = ${x}, y = ${y}`)
// 將會印出
//--> 5: x = 5, y = 2
最後的結果是很明顯的。
你可以測試這些程式碼並觀看結果,感謝 babel。
更多可用的資訊在 MDN。
特別感謝 @kurtextrem 的合作 :)!
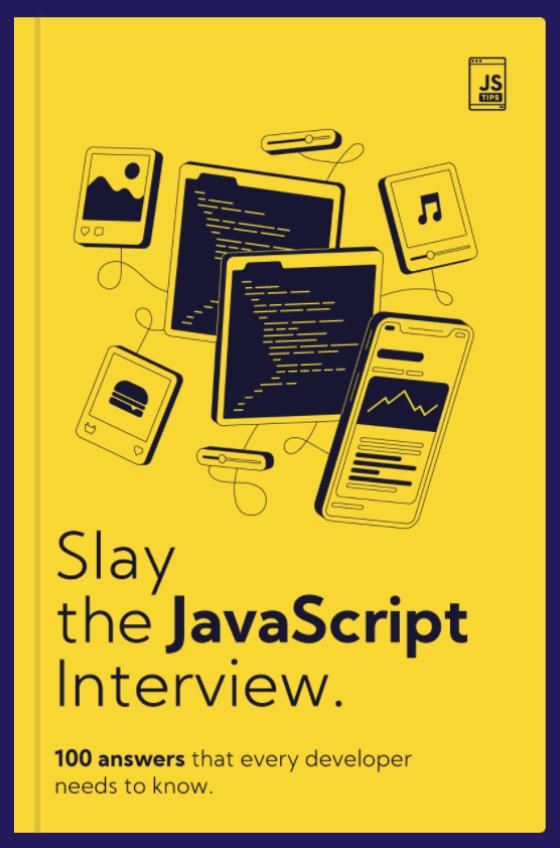
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
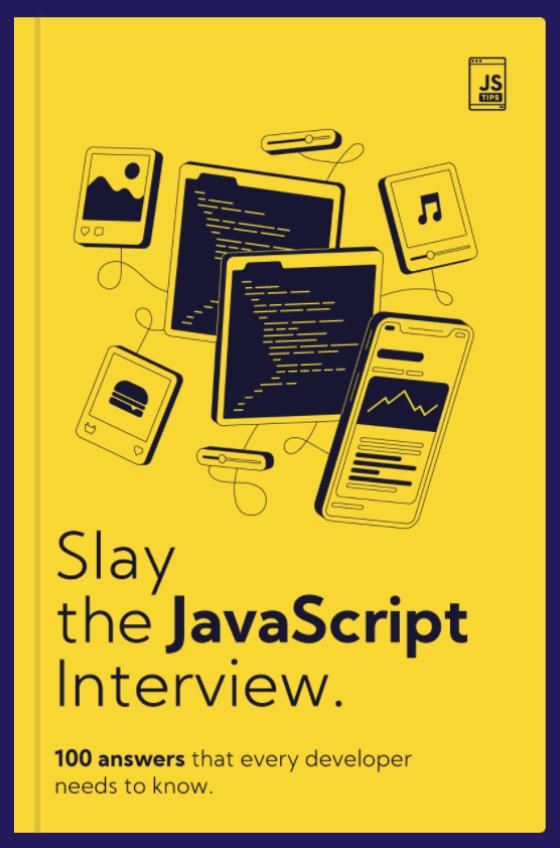
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW