在 JavaScript 中將多維陣列扁平化
三種不同的解決方法,將多維陣列合併為單一的陣列。
給定一個陣列:
var myArray = [[1, 2],[3, 4, 5], [6, 7, 8, 9]];
我們想到得到這個結果:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
方案一: 使用 concat()
和 apply()
var myNewArray = [].concat.apply([], myArray);
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
方案二:使用 reduce()
var myNewArray = myArray.reduce(function(prev, curr) {
return prev.concat(curr);
});
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
方案三:
var myNewArray3 = [];
for (var i = 0; i < myArray.length; ++i) {
for (var j = 0; j < myArray[i].length; ++j)
myNewArray3.push(myArray[i][j]);
}
console.log(myNewArray3);
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
在這裡觀察三種方式的實際應用。
方案四:使用 ES6 的展開運算符
var myNewArray4 = [].concat(...myArray);
console.log(myNewArray4);
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
在這裡 查看這四個方法的
如果需要無限的巢狀陣列嘗試使用 Lodash 的 flattenDeep()。
如果你擔心效能問題的話,這裡 有一個測試讓你來確認它們是如何執行的。
對於較大的巢狀陣列可以嘗試使用 Underscore 的 flatten()。
如果你好奇效能方面的表現, 這裡有相關的測試。
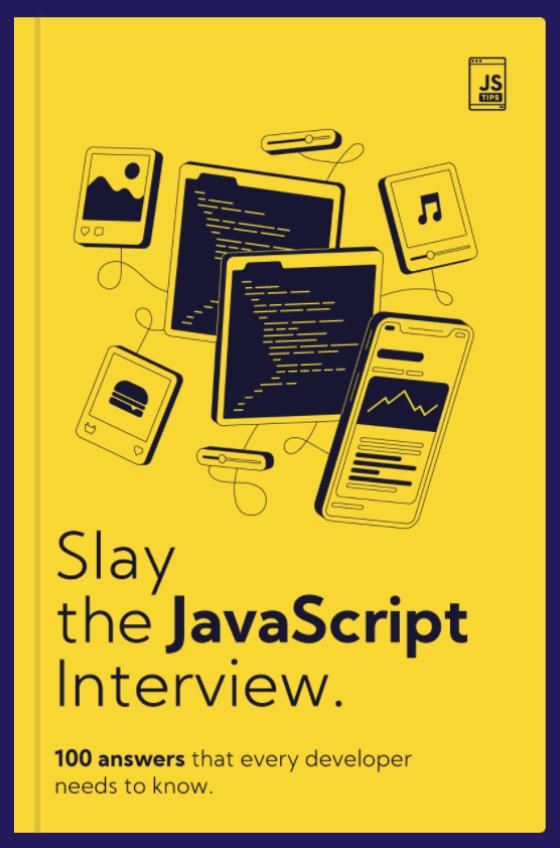
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
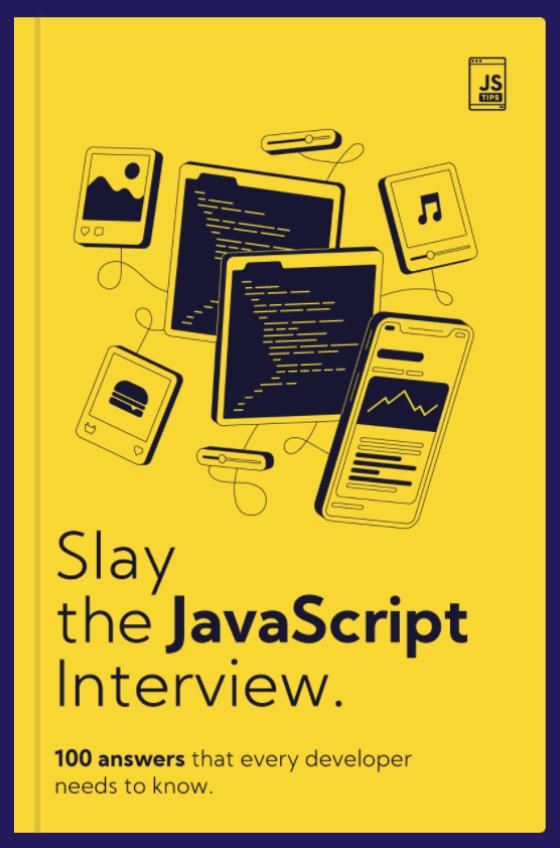
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW